TCAM Capacity
7280R2 Switches
# Get the devices that have the egw (EVPN gateway) tag
let devices = merge(`analytics:/tags/labels/devices/switch_role/value/egw/elements`)
# Get the L3 hardware capacity for all devices
let hwCapL3 =`*:/Sysdb/hardware/capacity/status/l3/entry`
# Get the devices that are part of a specific pod
let podDeviceList = merge(`analytics:/tags/labels/devices/pod_name/value/<_pod_name>/elements`)
# If the POD_NAME variable is not set (none) show the utilization for all pods
if str(_pod_name) == "" {
let filteredHwCapL3 = hwCapL3 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
} else {
let filteredHwCapL3 = hwCapL3 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}")) && dictHasKey(podDeviceList, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
}
for deviceKey, deviceValue in filteredHwCapL3{
filteredHwCapL3[deviceKey]["MAC"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LEM\", \"feature\": \"MAC\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LEM\", \"feature\": \"MAC\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["FEC Routing"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"FEC\", \"feature\": \"Routing\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"FEC\", \"feature\": \"Routing\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing1"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource1\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource1\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing2"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource2\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource2\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing3"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource3\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource3\"}")]["maxLimit"]*100
}
filteredHwCapL3 | map(_value | fields("MAC","FEC Routing", "Routing1", "Routing2", "Routing3"))
7050X3 Switches
# Get the devices that have the `hdl` (high-density leaf) tag
let devices = merge(`analytics:/tags/labels/devices/switch_role/value/hdl/elements`)
# Get the L3 hardware capacity for all devices
let hwCapL3 =`*:/Sysdb/hardware/capacity/status/l3/entry`
# Get the L2 hardware capacity for all devices
let hwCapL2 =`*:/Sysdb/hardware/capacity/status/l2/entry`
# Get the devices that are part of a specific pod
let podDeviceList = merge(`analytics:/tags/labels/devices/pod_name/value/<_pod_name>/elements`)
# If the POD_NAME variable is not set (none) show the utilization for all pods
if str(_pod_name) == "" {
let filteredHwCapL3 = hwCapL3 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
let filteredHwCapL2 = hwCapL2 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
} else {
let filteredHwCapL3 = hwCapL3 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}")) && dictHasKey(podDeviceList, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
let filteredHwCapL2 = hwCapL2 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}")) && dictHasKey(podDeviceList, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
}
# Build a new table with MAC, HOST, LPM and NextHop utilization
for deviceKey, deviceValue in filteredHwCapL3{
filteredHwCapL3[deviceKey]["Host Percent"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"Host\", \"feature\": \"\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"Host\", \"feature\": \"\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["LPM Percent"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LPM\", \"feature\": \"\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LPM\", \"feature\": \"\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["NextHop Percent"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"NextHop\", \"feature\": \"\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"NextHop\", \"feature\": \"\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["MAC Percent"] = filteredHwCapL2[deviceKey][complexKey("{\"chip\": \"Linecard0/0\", \"table\":\"MAC\", \"feature\": \"L2\"}")]["used"] / filteredHwCapL2[deviceKey][complexKey("{\"chip\": \"Linecard0/0\", \"table\":\"MAC\", \"feature\": \"L2\"}")]["maxLimit"]*100
}
filteredHwCapL3 | map(_value | fields("MAC Percent","Host Percent", "LPM Percent", "NextHop Percent"))
7020R Switches
# Get the devices that have the `ldl` (low-density leaf) tag
let devices = merge(`analytics:/tags/labels/devices/switch_role/value/ldl/elements`)
# Get the L3 hardware capacity for all devices
let hwCapL3 =`*:/Sysdb/hardware/capacity/status/l3/entry`
# Get the multicast hardware capacity for all devices
let hwCapMcast = `*:/Sysdb/hardware/capacity/status/mcast/entry`
# Get the devices that are part of a specific pod
let podDeviceList = merge(`analytics:/tags/labels/devices/pod_name/value/<_pod_name>/elements`)
let filteredHwCapL3 = hwCapL3 | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}")) && dictHasKey(podDeviceList, complexKey("{\"deviceID\": \""+_key+"\"}")))| map(merge(_value) )
let filteredHwCapMcast = hwCapMcast | where(dictHasKey(devices, complexKey("{\"deviceID\": \""+_key+"\"}")) && dictHasKey(podDeviceList, complexKey("{\"deviceID\": \""+_key+"\"}"))) | map(merge(_value) )
# Build a new table with MAC, MCDB, FEC Routing, Routing1, Routing2 and Routing3 utilization
for deviceKey, deviceValue in filteredHwCapL3{
filteredHwCapL3[deviceKey]["MAC"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LEM\", \"feature\": \"MAC\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"LEM\", \"feature\": \"MAC\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["FEC Routing"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"FEC\", \"feature\": \"Routing\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"\", \"table\":\"FEC\", \"feature\": \"Routing\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing1"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource1\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource1\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing2"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource2\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource2\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["Routing3"] = filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource3\"}")]["used"] / filteredHwCapL3[deviceKey][complexKey("{\"chip\": \"Jericho\", \"table\":\"Routing\", \"feature\": \"Resource3\"}")]["maxLimit"]*100
filteredHwCapL3[deviceKey]["MCDB"] = filteredHwCapMcast[deviceKey][complexKey("{\"chip\": \"Jericho0\", \"table\":\"MCDB\", \"feature\": \"\"}")]["used"] / filteredHwCapMcast[deviceKey][complexKey("{\"chip\": \"Jericho0\", \"table\":\"MCDB\", \"feature\": \"\"}")]["maxLimit"]*100
}
filteredHwCapL3 | map(_value | fields("MAC","MCDB","FEC Routing", "Routing1", "Routing2", "Routing3"))
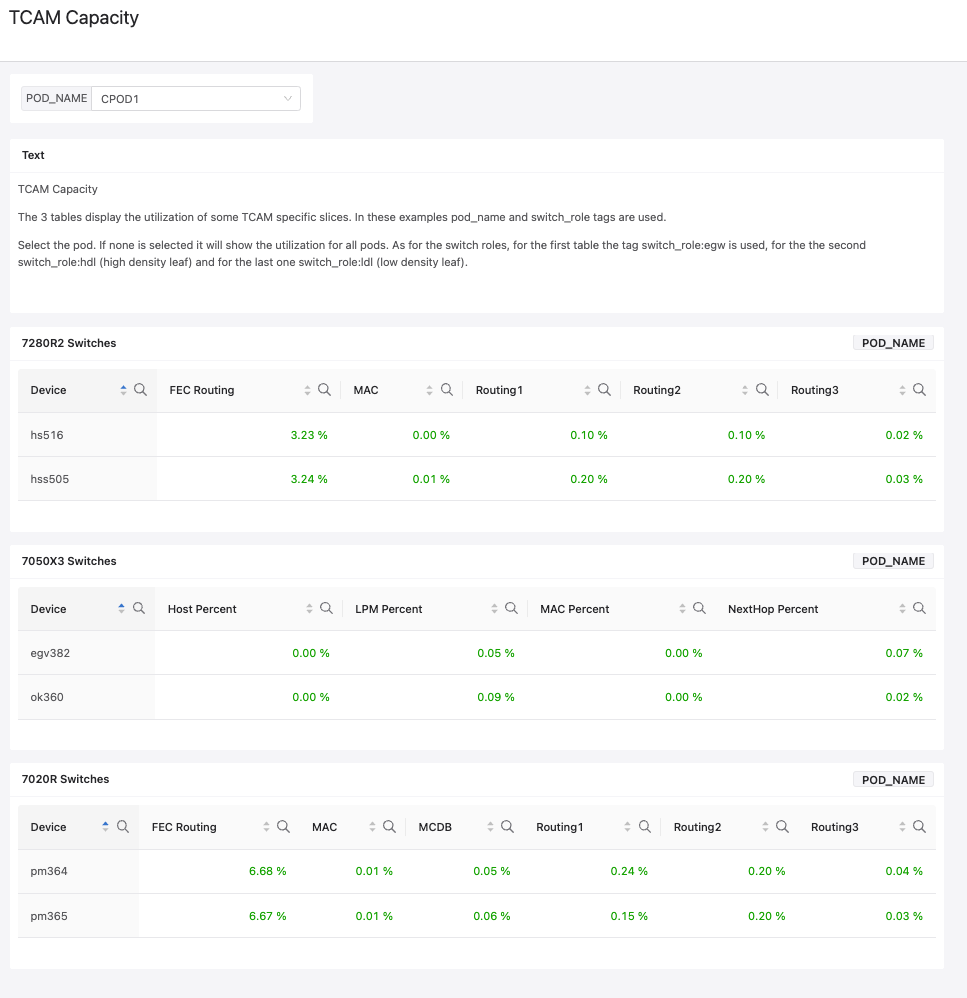